FLUTTER for Beginners - Ultimate Guide
January 22nd, 2021
In this day and age, we realize how integral mobile phones have become and how we rely on them on a day to day basis. It is nothing but evident that increasingly, if not all then most of the technologies and applications are starting to develop mobile applications to ensure that it reaches to the most number of people and becomes accessible to everyone.
To facilitate the needs of people, we see an ever increase in the number of mobile application developers. This blog is catered to the needs of those who want to test the waters of app development and get a hang of what it looks like.
Before delving into the basics of mobile application development, I'd like to say that it is not easy to grasp the concepts of mobile development from the get-go because of how convoluted and intermixed some of the processes are, hence feel free to use the internet and refer to articles which explain every component of flutter and dart in detail.
Essentially, the only prerequisite to learning flutter and getting ahead with app development is the installation of some applications like the android studio and visual studio code to act as an IDE for easier access and development. Along with that, we would need dart as the primary language to work with. To introduce dart, I'd like to say that it is an empowering client-optimized language for fast applications, irrespective of the platform a person uses.
Moving ahead with application development, it's imperative to understand the use of an IDE for the process. IDE stands for Integrated Development Environment. In essence, An Integrated Development Environment (IDE) is a software environment used to write other programs using tools like an editor and compiler.
This can prove to be an extremely useful tool when coding uses various languages for many reasons. Examples of different IDE's include Eclipse, Visual Studios, and NetBeans. Each IDE has its niche strengths and weaknesses. In my case, I chose Visual Studio because of the seamless integration it provides when used with the dart and how it harnesses the qualities of flutter and dart.
An alternative to this would be Android Studio which is equally efficient and accessible. It's only a matter of preference when it comes to the choice and selection of IDEs. Hence, choosing one and sticking with it would be recommended.
There exist multiple competitors and alternatives to flutter for app development, namely React Native, Xamarin, Java, Kotlin, etc. Now, we're to ask why we prefer flutter over the other alternatives.
To give a rough idea, I can say that Flutter provides better means of beautification, customization, UI/UX amongst many other advantages. Notably, flutter also gives us the option to work on a single code which would suffice for both android and ios based devices. The current development of flutter is on providing the ability to create desktop-based applications as well but that is only in its initial stages.
This is the image of a sample dart code and application that is pre-built when you launch a new project. Here, in essence, the code is just a counter to check how many times you have clicked on the + icon at the bottom right.
If you look closely, you'd notice a yellow lightning icon above the emulated phone. This function is exclusive to flutter known as "hot reload". This is yet another striking feature of flutter and gives it an edge over its competitors.
To explain hot reload in layman terms, it is a feature that lets you make instant changes in your code and see them being reflected in your application without it having to reload and relaunch completely.
This substantially affects the time that it takes to relaunch the application and sometimes can range to several minutes in case the development is of a huge program. If you're new to application development, you'd not realize the importance of this feature till you've once used it. Now, here are few basics of flutter.
First things first, we need to understand that management of code is one of the quintessential abilities a person must possess to separate them from the hoi-polloi. Given below is an example to understand how code should be differentiated.
The file is called "main. dart". This is usually the file that gets compiled during run-time and is the file that must contain an executable code that makes sure that you have a working application.
For citing an example, I have created another file here called "code. dart". This is just an example to reflect how a big piece of code can be converted into different chunks of code depending on the user and then be clubbed altogether in the main file to use as an executable.
Both the files, code.dart and main.dart serves the purpose of working as files where we can enter dart code to make an application. The difference lies in the fact that we have segregated one big chunk of code into 2 different parts to increase its readability and make it more efficient to debug the code in case of problems and bugs.
To get started with a simple application interface, we'll start by learning how the screen on your phone can be utilized as space for building different interfaces. Two main divisions that we're gonna create here are:
AppBar:
An app bar consists of a toolbar and potentially other widgets, such as a TabBar and a FlexibleSpaceBar. App bars typically expose one or more common actions with IconButtons which are optionally followed by a PopupMenuButton for less common operations.
Scaffold:
A scaffold is a class in flutter which provides many widgets or we can say APIs like Drawer, SnackBar, BottomNavigationBar, FloatingActionButton, AppBar, etc. The scaffold will expand or occupy the whole device screen. It will occupy the available space. The scaffold will provide a framework to implement the basic material design layout of the application.
This is a sample of one of the most basic mobile applications that can be created using flutter.
The step by step process would be,
First off, you see how I have divided the code into two files, the basic.dart file and the main.dart file. The main file contains the myapp() executable under the runApp() function to compile our code and make it function as an application.
The use of this very aspect was mentioned earlier, where I talked about how separating chunks of code into multiple files based on content increases the readability and accessibility of the file.
Moving along, we see that we have imported rather important libraries that are necessary to harness the flutter components in the dart.
Libraries essentially are collections of subprograms that are used for the development of an application or software. The main application is called "myapp". Everything we have done comes under this function. The myapp contains almost all of the relevant information that would be needed for the functioning of this application
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; myapp() { var shoppingIcon = Icon(Icons.add_shopping_cart); var alarmIcon = Icon(Icons.alarm); press1() { print("Clicked on the shopping button"); } press2() { print("Clicked on the alarm button"); } var shoppingButton = IconButton(icon: shoppingIcon, onPressed: press1); var alarmButton = IconButton(icon: alarmIcon, onPressed: press2); var body = Container( height: 500, width: 500, alignment: Alignment.bottomCenter, child: Column( children: [shoppingIcon, alarmIcon], ), decoration: BoxDecoration( color: Colors.yellow, borderRadius: BorderRadius.circular(20.0)), ); var url = 'https://media-exp1.licdn.com/dms/image/C5103AQHVwga7aAEf6g/profile-displayphoto-shrink_400_400/0?e=1600905600&v=beta&t=j5A71sg_BGWXSA6ifzORN4CiMYcxo9fxHDQxDHttBIQ'; var MyImage = Image.network(url); var appBar = AppBar( title: Center(child: Text('Hello there!')), backgroundColor: Colors.greenAccent, actions: [shoppingButton, alarmButton], leading: MyImage, ); var myHome = Scaffold( appBar: appBar, body: body, ); var design = MaterialApp( home: myHome, debugShowCheckedModeBanner: false, ); return design; }
Now, as I shared the entire code with you, we can go over it step by step. Essentially we need to understand AppBar, Scaffold, and MaterialApp first. To explain these, I have hyperlinked the individual explanations which can be referred to.
In essence, AppBar() looks something like the top green bar in the emulated phone, the main body looks like the yellow region in the emulation and all of this is a feature under Scaffold which in turn is a child of MaterialApp(). At once it sounds like a lot of inflow of information, but if you take it to step by step, it's easy to use!
Going over the other parts of the code, We can see some of the keywords such as myHome, design, etc. These are variables that hold values and can be later used to display various functionalities on the screen. The words in green are pre-existing functions that serve various purposes in the application development aspect. For example, the Icon() is a pack that contains the size, alignment, picture, and orientations of the icons a person would want to use in his application.
In the end, I'd like to give you a small task. Create a basic flutter application that takes in an image of yours from a URL and use that image as your body in the application with the app bar titled "My image"
ABOUT THE AUTHOR
Siddharth is an undergrad student pursuing Computer Sciences at SRM Institute of Science and Technology. He is a cybersecurity researcher with an intermediate background in penetration testing, network security, machine learning, deep learning and mobile application development. His primary areas of work include the enhancement of home security systems and effective integration of smart grids with blockchain. For further information or assistance, you can contact him at singhsiddharth820@gmail.com
Insight Categories
Alumni Speaks
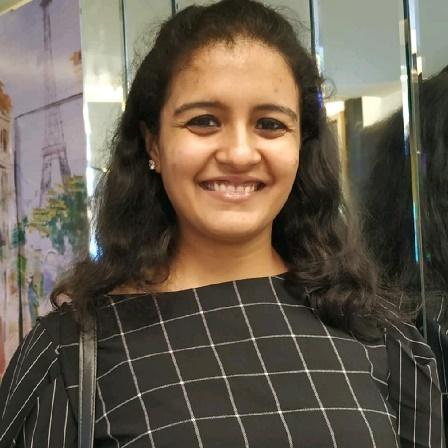
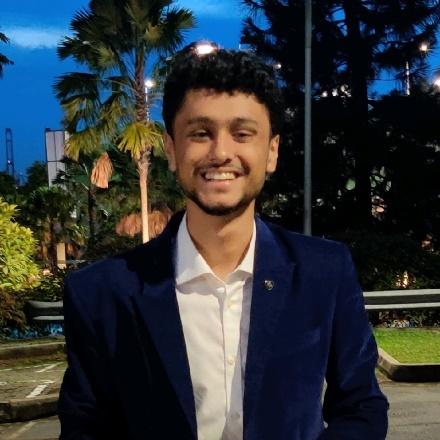
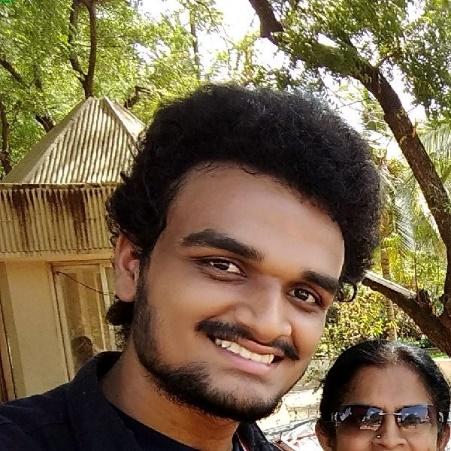
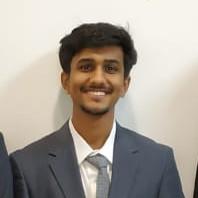
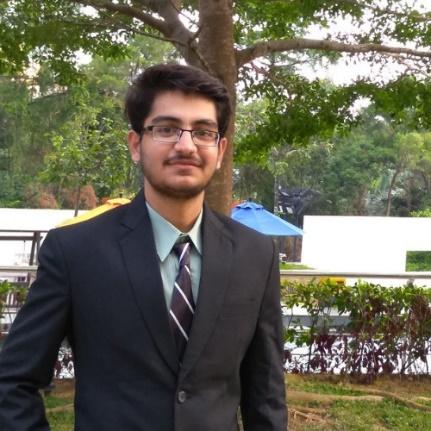
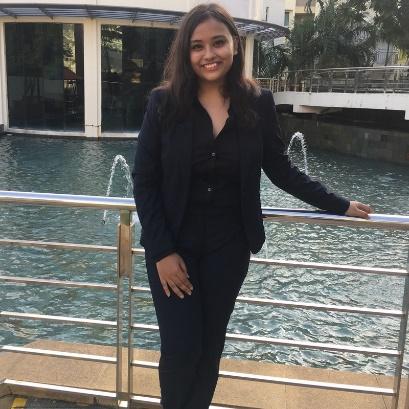
It started off in a more hectic manner than I could expect. ... read more
- Priyanshi Somani, Manipal Institute of Technology
“GAIP is perfectly aligned with someone's goal who wishes to experience an outburst of academic challenges while working on projec ... read more
- Sukriti Shaw, SRM Institute of Science and Technology
“Combining different characters and skillset from different institutes and domains in a new country and fantastic institute, it wa ... read more
- Shaolin Kataria, VIT, Vellore
“An enriching and enthralling experience. The course was extensive but worth every penny. ... read more
- Arudhra Narasimhan V, SASTRA DEEMED TO BE UNIVERSITY
“I personally learned quite a bit here but the 6-month project or LOR aren't as easy to get as was portrayed before. ... read more
- Dwait Bhatt, BITS PILANI
“It was a great experience for me, and far beyond my expectations. ... read more
- Shrikant Tarwani, LNM Institute of Information Technology
“This Internship is the perfect balance of theory and practical application. ... read more
- Mahima Borah, Manipal Institute of Technology
“This Internship has strengthened my concepts on Artificial Intelligence and Deep learning which are the hot words of today’s t ... read more
- Mansi Agarwal, Delhi Technological University